A simple explanation of the four basic concepts of Object-Oriented Programming (OOP)
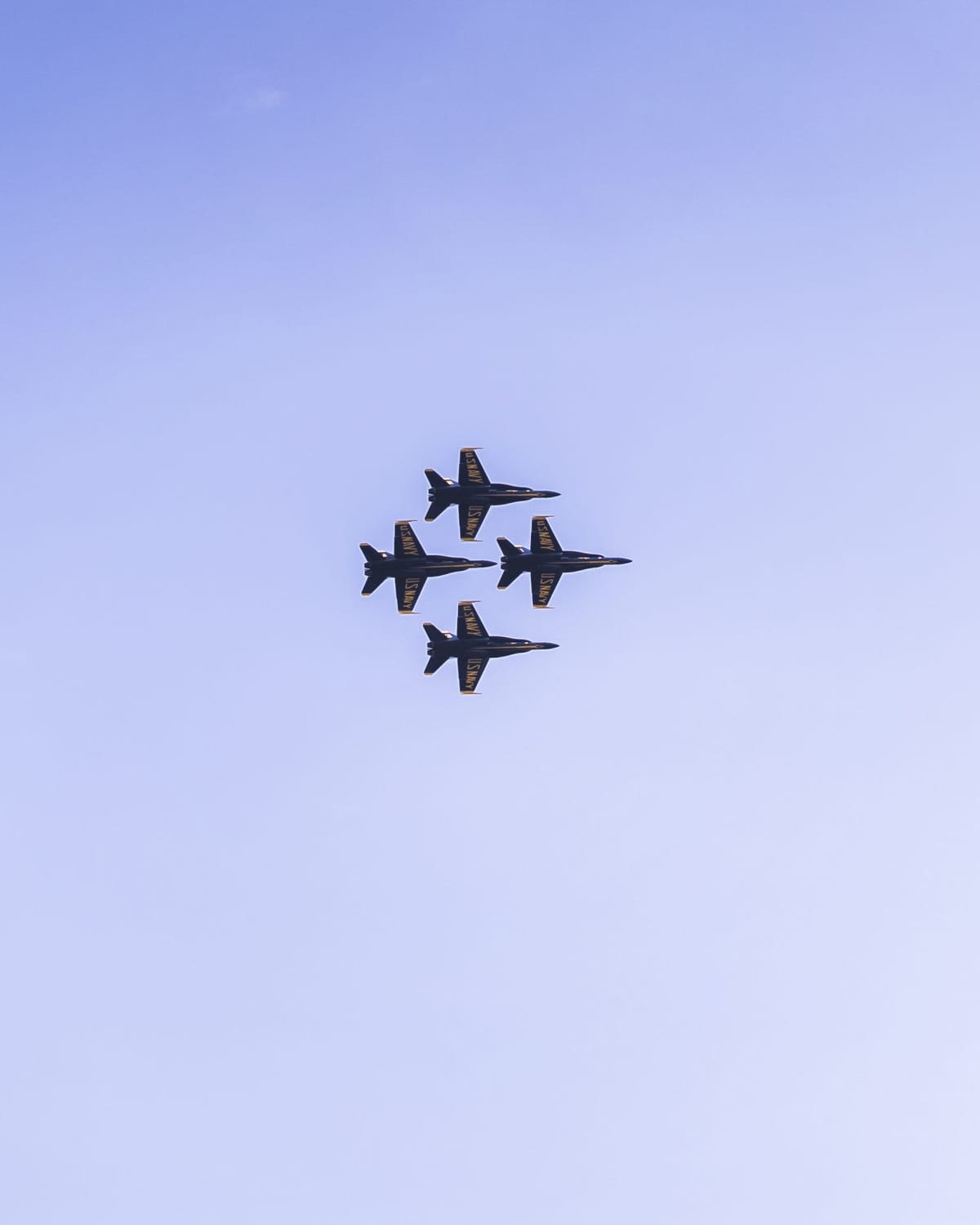
As a software engineer, the concept of object-oriented programming is something you need to learn, understand, and be able to explain simply to anyone who asks. Therefore, most interviews for programming jobs will include questions about object-oriented programming.
In this post, I’d like to share a list and a simple description of the four concepts of object-oriented programming.
The four principles of object-oriented programming are encapsulation, abstraction, inheritance, and polymorphism.
Encapsulation
It means that a group of related properties, methods, and other members are part of a single unit or object. In other words, it’s like a package of goods where all of the items are used and needed for one purpose. It’s like going to an all-inclusive resort in Mexico that includes everything with one payment and in one place. A single package includes your hotel room, entertainment, food, gym, the beach, etc.
Abstraction
It is the principle used to describe how a program handles complexity by hiding the implementation details and displaying only essential features to a user. So, for example, you can flip a switch on your wall and see that the lights come up without knowing how electricity works. Likewise, you order things online, pay by entering a number, and these things show up at your door, all without you knowing how these things operate behind the scenes – all of these are real-life examples of abstractions.
Inheritance
It enables you to create a new class that reuses, extends, and modifies the behavior defined in another class.
The class whose members are inherited is called the base class, and the class that inherits those members is called the derived class.
In C#, all classes implicitly inherit from the Object class.
A real-life example of inheritance could be vehicles as the base class, and then we can have things like motorized and non-motorized vehicles as derived classes. The idea is that the base class can have generic properties (for example, all vehicles in this example have wheels) that can be applied and used by all derived classes, and then each derived class can have its own properties and behavior depending on its need.
- Vehicles
- Motorized
- Car
- Bus
- Non-motorized
- Bicycle
- Cart
- Rickshaw
- Motorized
Polymorphism
It means that you can have multiple classes and use them interchangeably, even though each class implements the same properties or methods in different ways. This gives you the ability to create more modular and extensible applications. With polymorphism, the program will call a specific method determined at runtime based on the type of the object.
A real-life example of this is blood groups. Humans all share many of the same characteristics, but we also have unique characteristics within a population, such as blood type. Human blood groups are examples of genetic polymorphism.
If you are interested in learning more about object-oriented programming, I recommend the books below:
- Design Patterns: Elements of Reusable Object-Oriented Software
- Head First Object-Oriented Analysis and Design